Hive Developer Portal
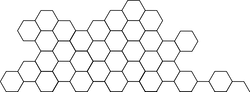
Submit Post
This example will broadcast a new post to the blockchain using the values provided.
Full, runnable src of Submit Post can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-10_submit_post.zip).
In this tutorial will explain and show you how to submit a new post to the Hive
blockchain using the commit
class found within the beem library.
Intro
The beem library has a built-in function to transmit transactions to the blockchain. We are using the transactionbuilder
in the the library. It should be noted that comments and new post are both treated as the comment
operation with the only difference being that a comment/reply has an additional parameter containing the parent_author
/parent_permlink
that corresponds to the original post/comment.
- author - The account that you are posting from
- title - The title of the post
- body - The body of the post
- permlink - A unique identifier, scoped to author
- parent_author - Empty string (for posts) or author being replied to (for replies)
- parent_permlink - Category (for posts) or permlink being replied to (for replies)
- json_metadata - JSON meta object that can be attached to the post
- tags - Between 1 and 5 key words that defines the post
We will only be using the above parameters as these are the only ones required to create a basic post. If you want to explore the other parameters further you can find more information HERE.
Also see:
Steps
- App setup - Library install and import. Connection to Hive node
- Variable input and format - Input and creation of varialbes
- Post submission and result - Committing of transaction to the blockchain
1. App setup
In this tutorial we use the following packages:
random
andstring
- used to create a random string used for thepermlink
getpass
- capture wif without showing it on the screenjson
- generatejson_metadata
beem
- hive library and interaction with Blockchain
We import the libraries, connect to your local testnet
, and initialize the Hive class.
import random
import string
import getpass
import json
from beem import Hive
from beem.transactionbuilder import TransactionBuilder
from beembase.operations import Comment
Because this tutorial alters the blockchain we have to connect to the testnet. We also require the private posting key
of the contributing author in order to commit the post which is why we’re using a testnet
node.
2. Variable input and format
The first three variables are captured via a simple string input while the tags
variable is captured in the form of an array.
#capture variables
author = input('Username: ')
title = input('Post Title: ')
body = input('Post Body: ')
#capture list of tags and separate by " "
taglimit = 2 #number of tags 1 - 5
taglist = []
for i in range(1, taglimit+1):
print(i)
tag = input(' Tag : ')
taglist.append(tag)
The tags
parameter needs to be formatted within the json_metadata
field as JSON. We also use a random generator to create a new permlink
for the post being created.
#random generator to create post permlink
permlink = ''.join(random.choices(string.digits, k=10))
The random generator is limited to 10 characters in this case but the permlink can be up to 256 bytes. The permlink is unique to the author only which means that multiple authors can have the same permlink for the their post.
3. Post submission and result
The last step is to transmit the post through to the blockchain. All the defined parameters are signed and broadcasted. We also securely prompt for the posting key right before signing.
# client = Hive('https://testnet.openhive.network') # Public Testnet
client = Hive('http://127.0.0.1:8090') # Local Testnet
tx = TransactionBuilder(blockchain_instance=client)
tx.appendOps(Comment(**{
"parent_author": '',
"parent_permlink": taglist[0], # we use the first tag as the category
"author": author,
"permlink": permlink,
"title": title,
"body": body,
"json_metadata": json.dumps({"tags": taglist})
}))
wif_posting_key = getpass.getpass('Posting Key: ')
tx.appendWif(wif_posting_key)
signed_tx = tx.sign()
broadcast_tx = tx.broadcast(trx_id=True)
print("Post created successfully: " + str(broadcast_tx))
A simple confirmation is printed on the screen if the post is committed successfully.
You can also check on your local testnet using database_api.find_comments for the post.
Final code:
import random
import string
import getpass
import json
from beem import Hive
from beem.transactionbuilder import TransactionBuilder
from beembase.operations import Comment
#capture variables
author = input('Username: ')
title = input('Post Title: ')
body = input('Post Body: ')
#capture list of tags and separate by " "
taglimit = 2 #number of tags 1 - 5
taglist = []
for i in range(1, taglimit+1):
print(i)
tag = input(' Tag : ')
taglist.append(tag)
#random generator to create post permlink
permlink = ''.join(random.choices(string.digits, k=10))
# client = Hive('https://testnet.openhive.network') # Public Testnet
client = Hive('http://127.0.0.1:8090') # Local Testnet
tx = TransactionBuilder(blockchain_instance=client)
tx.appendOps(Comment(**{
"parent_author": '',
"parent_permlink": taglist[0], # we use the first tag as the category
"author": author,
"permlink": permlink,
"title": title,
"body": body,
"json_metadata": json.dumps({"tags": taglist})
}))
wif_posting_key = getpass.getpass('Posting Key: ')
tx.appendWif(wif_posting_key)
signed_tx = tx.sign()
broadcast_tx = tx.broadcast(trx_id=True)
print("Post created successfully: " + str(broadcast_tx))
To Run the tutorial
You can launch a local testnet, with port 8090 mapped locally to the docker container:
docker run -d -p 8090:8090 inertia/tintoy:latest
For details on running a local testnet, see: Setting Up a Testnet
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/10_submit_post
pip install -r requirements.txt
python index.py
- After a few moments, you should see a prompt for input in terminal screen.