Hive Developer Portal
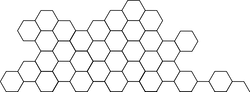
Power Up Hive
Power up an account’s Hive using either Hive Signer or a client-side signing.
Full, runnable src of Power Up Hive can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-24_power_up_hive.zip).
In this tutorial we show you how to check the HIVE balance of an account on the Hive blockchain and how to power up your HIVE into HIVE Power using the commit
class found within the beem library.
Intro
The beem library has a built-in function to transmit transactions to the blockchain. We are using the transfer_to_vesting
method found within the account instance. When you power up you convert your HIVE into HIVE Power to increase your influence on Hive. Before we do the conversion, we use the Account
function to check the current HIVE balance of the account to see what is available to power up. This is not strictly necessary but adds to the usability of the process. The transfer_to_vesting
method has 2 parameters:
- amount - The amount of HIVE to power up. This must be of the
float
data type - to - The account to where the HIVE will be powered up
Also see:
Steps
- App setup - Library install and import. Connection to testnet
- User information and Hive node - Input user information and connection to Hive node
- Check balance - Check current vesting balance of user account
- Conversion amount - Input power up amount and check valid transfer
- Commit to blockchain - Commit transaction to blockchain
1. App setup
In this tutorial we use 2 packages:
beem
- hive library and interaction with Blockchainpick
- helps select the query type interactively
We import the libraries and connect to the testnet
.
import pprint
from pick import pick
import getpass
from beem import Hive
from beem.account import Account
Because this tutorial alters the blockchain we connect to a testnet so we don’t create spam on the production server.
2. User information and Hive node
We require the private active key
of the user in order for the conversion to be committed to the blockchain. This is why we are using a testnet. The values are supplied via the terminal/console before we initialize the beem class.
# capture user information
account = input('Enter username: ')
wif_active_key = getpass.getpass('Enter private ACTIVE key: ')
# node_url = 'https://testnet.openhive.network' # Public Testnet
node_url = 'http://127.0.0.1:8090' # Local Testnet
# connect node and private active key
client = Hive(node_url, keys=[wif_active_key])
3. Check balance
In order to give the user enough information to make the conversion we check the current balance of the account using the Account
instance.
# check valid user and get account balance
account = Account(account, blockchain_instance=client)
balance = account['balance']
symbol = balance.symbol
print('Available balance: ' + str(balance) + '\n')
input('Press any key to continue')
The results of the query are displayed in the console/terminal.
4. Conversion amount
Both the amount
and the to
parameters are assigned via input from the terminal/console. The user is given the option to power up the HIVE to their own account or to another user’s account. The amount has to be greater than zero and no more than the total available HIVE of the user.
# choice of account
title = 'Please choose an option for an account to power up: '
options = ['SELF', 'OTHER']
# get index and selected transfer type
option, index = pick(options, title)
if (option == 'OTHER') :
# account to power up to
to_account = input('Please enter the ACCOUNT to where the ' + symbol + ' will be powered up: ')
to_account = Account(to_account, blockchain_instance=client)
else :
print('\n' + 'Power up ' + symbol + ' to own account' + '\n')
to_account = account
# amount to power up
amount = float(input('Please enter the amount of ' + symbol + ' to power up: ') or '0')
5. Commit to blockchain
Now that all the parameters have been assigned we can continue with the actual transmission to the blockchain. The output and commit is based on the validity of the amount that has been input.
# parameters: amount, to, account
if (amount == 0) :
print('\n' + 'No ' + symbol + ' entered for powering up')
exit()
if (amount > balance) :
print('\n' + 'Insufficient funds available')
exit()
account.transfer_to_vesting(amount, to_account.name)
print('\n' + str(amount) + ' ' + symbol + ' has been powered up successfully to ' + to_account.name)
The result of the power up transfer is displayed on the console/terminal.
As an added check we also display the new HIVE balance of the user on the terminal/console.
# get new account balance
account.refresh()
balance = account['balance']
print('New balance: ' + str(balance))
Final code:
import pprint
from pick import pick
import getpass
from beem import Hive
from beem.account import Account
# capture user information
account = input('Enter username: ')
wif_active_key = getpass.getpass('Enter private ACTIVE key: ')
# node_url = 'https://testnet.openhive.network' # Public Testnet
node_url = 'http://127.0.0.1:8090' # Local Testnet
# connect node and private active key
client = Hive(node_url, keys=[wif_active_key])
# check valid user and get account balance
account = Account(account, blockchain_instance=client)
balance = account['balance']
symbol = balance.symbol
print('Available balance: ' + str(balance) + '\n')
input('Press any key to continue')
# choice of account
title = 'Please choose an option for an account to power up: '
options = ['SELF', 'OTHER']
# get index and selected transfer type
option, index = pick(options, title)
if (option == 'OTHER') :
# account to power up to
to_account = input('Please enter the ACCOUNT to where the ' + symbol + ' will be powered up: ')
to_account = Account(to_account, blockchain_instance=client)
else :
print('\n' + 'Power up ' + symbol + ' to own account' + '\n')
to_account = account
# amount to power up
amount = float(input('Please enter the amount of ' + symbol + ' to power up: ') or '0')
# parameters: amount, to, account
if (amount == 0) :
print('\n' + 'No ' + symbol + ' entered for powering up')
exit()
if (amount > balance) :
print('\n' + 'Insufficient funds available')
exit()
account.transfer_to_vesting(amount, to_account.name)
print('\n' + str(amount) + ' ' + symbol + ' has been powered up successfully to ' + to_account.name)
# get new account balance
account.refresh()
balance = account['balance']
print('New balance: ' + str(balance))
To Run the tutorial
You can launch a local testnet, with port 8090 mapped locally to the docker container:
docker run -d -p 8090:8090 inertia/tintoy:latest
For details on running a local testnet, see: Setting Up a Testnet
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/24_power_up_hive
pip install -r requirements.txt
python index.py
- After a few moments, you should see a prompt for input in terminal screen.