Hive Developer Portal
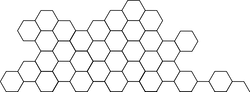
Grant Posting Permission
How to grant and revoke posting permission to another user.
Full, runnable src of Grant Posting Permission can be downloaded as part of: tutorials/python (or download just this tutorial: devportal-master-tutorials-python-30_grant_posting_permission.zip).
In this tutorial we show you how to check if someone has posting permission for an account on the Hive blockchain and how to grant or revoke that permission using the beem library.
Providing another user posting permission for your account can be used to allow multiple users to submit posts on a single hive community. Peakd is an example of such a community, as well as others, that allow you to schedule posts by automatically publishing on your behalf.
Intro
The beem library has a built-in function to transmit transactions to the blockchain. We are using the allow
and disallow
methods found within the Account
instance. Before we grant or revoke permission, we use the Account
module to check whether the requested user already has that permission or not. This is not strictly necessary but adds to the usability of the process.
The disallow
method uses the same process except for weight
which is not required.
There is a permission limit defined by HIVE_MAX_AUTHORITY_MEMBERSHIP
that limits the number of authority membership to 40 (max).
Also see:
Steps
- App setup - Library install and import. Input user info and connection to production
- Username validation - Check validity of user and foreign account
- Check permission status - Check current permission status of foreign account
- Commit to blockchain - Commit transaction to blockchain
1. App setup and connection
In this tutorial we use 2 packages:
beem
- hive library and interaction with Blockchainpick
- helps select the query type interactively
We import the libraries for the application.
from pick import pick
import getpass
from beem import Hive
from beem.account import Account
We require the private active key
of the user in order for the allow
or disallow
to be committed to the blockchain. The values are supplied via the terminal/console before we initialize the beem class with the supplied private key included.
# capture user information
account = input('Enter username: ')
wif_active_key = getpass.getpass('Enter private ACTIVE key: ')
# node_url = 'https://testnet.openhive.network' # Public Testnet
node_url = 'http://127.0.0.1:8090' # Local Testnet
# connect to production server with active key
client = Hive(node_url, keys=[wif_active_key])
2. Username validation
Both the main account granting the permission and the account that permission is being granted to are first checked to make certain that they do in fact exist. We do this with the Account
module.
# check valid user
account = Account(account, blockchain_instance=client)
print('Current posting authorizations: ' + str(account['posting']['account_auths']))
# get account to authorise and check if valid
foreign = input('Please enter the account name for POSTING authorization: ')
if (foreign == account.name):
print('Cannot allow or disallow posting permission to your own account')
exit()
foreign = Account(foreign, blockchain_instance=client)
3. Check permission status
In order to determine which function to execute (allow
or disallow
) we first need to check whether the requested user already has permission or not. We do this with the same variable created in the previous step. The Account
module has a value - posting
- that contains an array of all the usernames that has been granted posting permission for the account being queried. We use this check to limit the options of the user as you cannot grant permission to a user that already has permission or revoke permission of a user that does not yet have permission. The information is displayed on the options list.
# check if foreign account already has posting auth
title = ''
for auth in account['posting']['account_auths']:
if (auth[0] == foreign.name):
title = (foreign.name + ' already has posting permission. Please choose option from below list')
options = ['DISALLOW', 'CANCEL']
break
if (title == ''):
title = (foreign.name + ' does not yet posting permission. Please choose option from below list')
options = ['ALLOW', 'CANCEL']
4. Commit to blockchain
Based on the check in the previous step, the user is given the option to allow
, disallow
or cancel
the operation completely. All the required parameters have already been assigned via console/terminal input and based on the choice of the user the relevant function can be executed. A confirmation of the successfully executed action is displayed on the UI.
option, index = pick(options, title)
if (option == 'CANCEL'):
print('operation cancelled')
exit()
if (option == 'ALLOW'):
account.allow(foreign=foreign.name, weight=1, permission='posting', threshold=1)
print(foreign.name + ' has been granted posting permission')
else:
account.disallow(foreign=foreign.name, permission='posting', threshold=1)
print('posting permission for ' + foreign.name + ' has been removed')
Final code:
from pick import pick
import getpass
from beem import Hive
from beem.account import Account
# capture user information
account = input('Enter username: ')
wif_active_key = getpass.getpass('Enter private ACTIVE key: ')
# node_url = 'https://testnet.openhive.network' # Public Testnet
node_url = 'http://127.0.0.1:8090' # Local Testnet
# connect with active key
client = Hive(node_url, keys=[wif_active_key])
# check valid user
account = Account(account, blockchain_instance=client)
print('Current posting authorizations: ' + str(account['posting']['account_auths']))
# get account to authorise and check if valid
foreign = input('Please enter the account name for POSTING authorization: ')
if (foreign == account.name):
print('Cannot allow or disallow posting permission to your own account')
exit()
foreign = Account(foreign, blockchain_instance=client)
# check if foreign account already has posting auth
title = ''
for auth in account['posting']['account_auths']:
if (auth[0] == foreign.name):
title = (foreign.name + ' already has posting permission. Please choose option from below list')
options = ['DISALLOW', 'CANCEL']
break
if (title == ''):
title = (foreign.name + ' does not yet posting permission. Please choose option from below list')
options = ['ALLOW', 'CANCEL']
option, index = pick(options, title)
if (option == 'CANCEL'):
print('operation cancelled')
exit()
if (option == 'ALLOW'):
account.allow(foreign=foreign.name, weight=1, permission='posting', threshold=1)
print(foreign.name + ' has been granted posting permission')
else:
account.disallow(foreign=foreign.name, permission='posting', threshold=1)
print('posting permission for ' + foreign.name + ' has been removed')
To Run the tutorial
You can launch a local testnet, with port 8090 mapped locally to the docker container:
docker run -d -p 8090:8090 inertia/tintoy:latest
For details on running a local testnet, see: Setting Up a Testnet
- review dev requirements
git clone https://gitlab.syncad.com/hive/devportal.git
cd devportal/tutorials/python/30_grant_posting_permission
pip install -r requirements.txt
python index.py
- After a few moments, you should see a prompt for input in terminal screen.